Optimize Your Rails Project with Memoization and “||=”
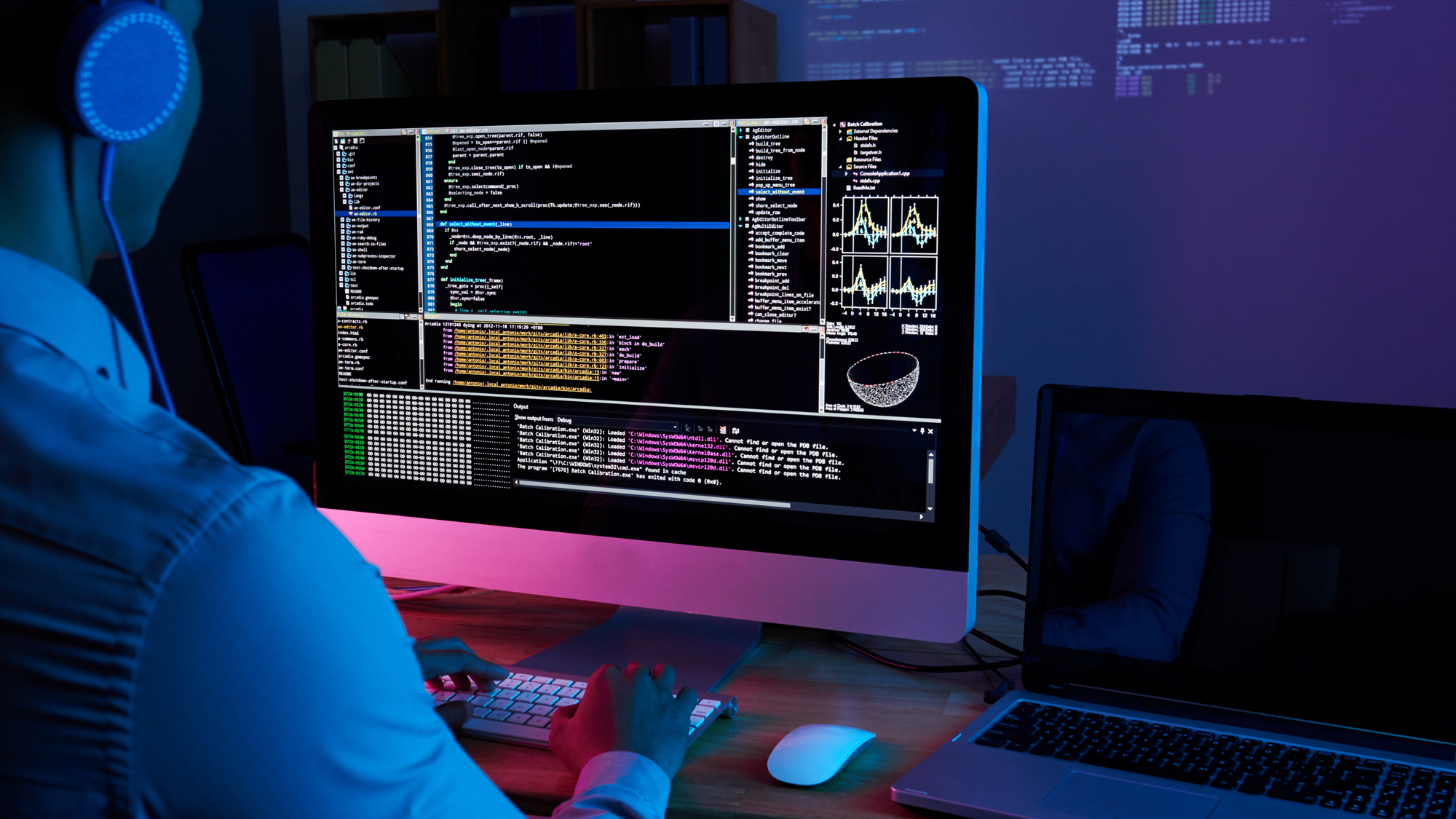
Memoization is an optimization technique that can significantly improve the result of expensive computation by caching the data. This technique works perfectly for Ruby on Rails applications. It includes caching the output of complex computing methods and returning it when the same input is entered again.
The ||= operator is a frequently used technique in Ruby memoization implementation.
Performance optimization is essential for Ruby on Rails applications, particularly when handling costly computations and a high volume of database queries. Memoization is a strategy that, by caching the outcomes of costly operations, can greatly increase performance. Using the ||= operator is one of the easiest ways to do memoization in Ruby.
Example
Let's take an example for calculating the factorial of a number. Factorial is denoted as (!n). It is calculated as the product of all positive integers less than or equal to n.
class Factorial
def calculate(n)
return 1 if n<=1
n * calculate(n - 1)
end
end
fact = Factorial.new
puts fact.calculate(5)
#output = 120
This is the example for calculating the factorial of a number. In this, each call to the calculation method leads to unnecessary calculation.
Challenges
The primary difficulty in this case is doing the same calculations again, which could cause impactful performance issues for greater values of n.
The solution for the above problem is Memoization with ‘||=’
Memoization is an approach where you actually save the result of a method that is being called repeatedly and then reuse the result of that stored method when that method is called again. The ‘||=’ operator in Ruby provides a simple way to implement memoization.
‘||=’ this operator is shorthand for “or equals”. It assigns a value to a variable only if that variable is nil or false.
Example
With Memoization
class Factorial
def initialize
@value = {}
end
def calculate(n)
return 1 if n <= 1
if @value[n]
puts "Returning from memoized value for #{n}!"
return @value[n]
end
puts "Calculating factorial for #{n}"
@value[n] ||= n * calculate(n - 1)
end
end
fact = Factorial.new
puts fact.calculate(5)
#output
puts fact.calculate(5)
Calculating factorial for 5
Calculating factorial for 4
Calculating factorial for 3
Calculating factorial for 2
120
puts fact.calculate(7)
Calculating factorial for 7
Calculating factorial for 6
Returning from memoized value for 5!
5040
puts fact.calculate(5)
Returning from memoized value for 5!
120
puts fact.calculate(3)
Returning from memoized value for 3!
6
In this example, the calculate method uses memoization with this ‘||=’ operator. The purpose of this operator is to check if the factorial for the given number is already present or not in the @value hash, if present then returns the prints and return value. If you first calculate 5! Then 7! Then memoization will use 5! Result for calculation, which is more efficient.
The above example prints the result with a memoized value.
Tips for Effective Memoization
- Use memoization for the methods that do complex computation or database queries and that function is being called frequently with the same input or slight change in the input. For instance, operations involving complex calculations, database queries, or API calls can benefit greatly from memoization.
- Ensure that the methods return the same result for the same inputs.
- Memoization stores the results in the memory. Which can lead to high memory usage if not managed properly. If a method has multiple possible inputs, then ensure that cache results do not take excessive memory. Consider limiting cache size.
- Memoization works best when the input is simple and immutable.
- Memoize at the lowest possible level of abstraction where the expensive operation occurs. Memoizing high-level methods might cache more data than necessary, leading to inefficiency.
- Document why and where memoization is being used in your code. This helps other developers understand the purpose and potential pitfalls, like cache invalidation scenarios.
Conclusion
Memoization with the ‘||=’ operator is a simple and powerful technique to optimize the performance of your applications. By caching the results of expensive operations, you can significantly reduce the computational overhead and improve the efficiency of your application.
Frequently Asked Questions?
1. What is memoization in Ruby?
Memoization is an optimization technique that caches expensive computation results, returning stored values for repeated inputs to improve performance.
2. How does the ||= operator work in Ruby memoization?
The ||= operator assigns a value to a variable only if it's nil or false, enabling simple memoization implementation.
3. When should I use memoization in Rails?
Use memoization for frequently called methods with complex computations or database queries that return consistent results for the same inputs.