Pinecone Vector DB Guide: Core Concepts Explained
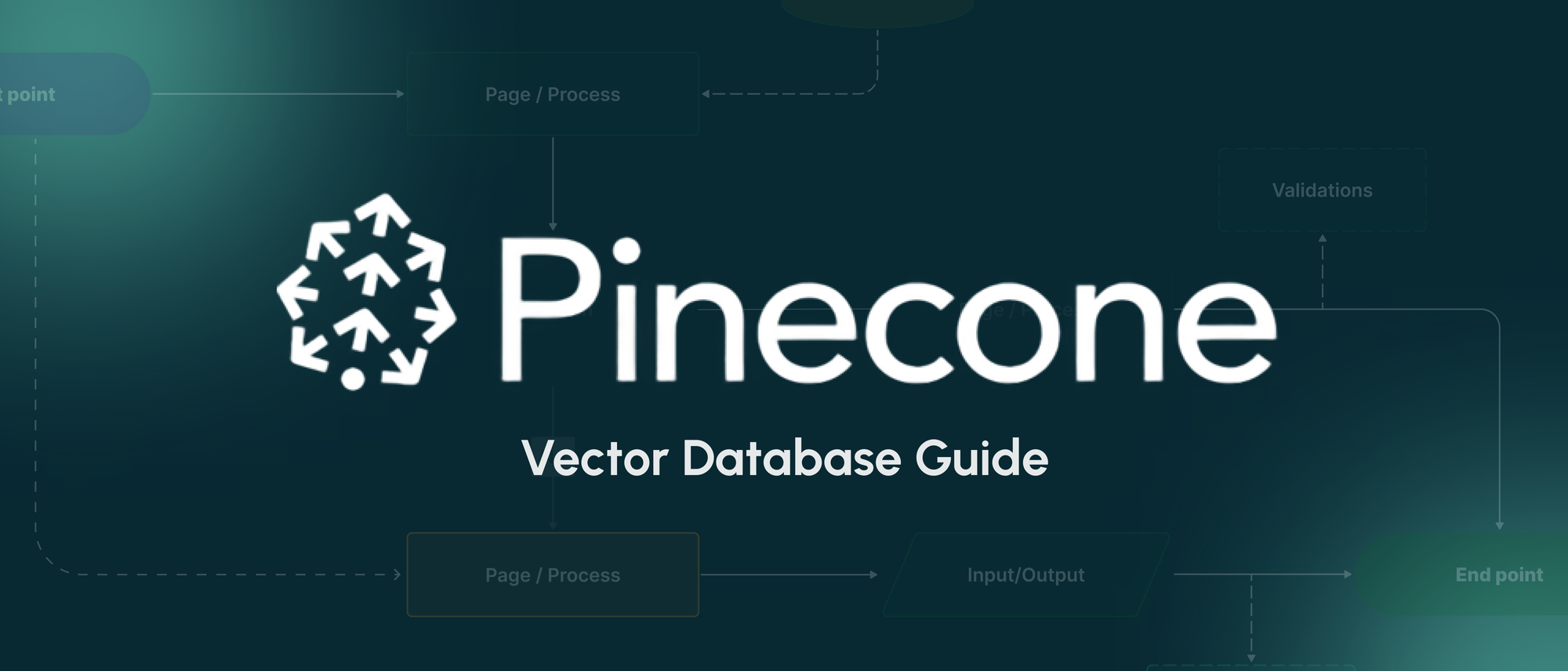
Think of AI as a super-smart library that needs to understand and remember massive amounts of information. But here's the challenge: how do we help AI organize and quickly find exactly what it needs? Enter Pinecone - imagine it as an AI's personal librarian that's incredibly fast at organizing and finding information.
Pinecone provides a managed vector database that enables developers to store, search, and retrieve high-dimensional vector embeddings efficiently. This blog will explore key concepts in Pinecone: chunks, embeddings, indexes, and namespaces. Understanding these components is essential for harnessing the full potential of Pinecone.
What are Chunks?
Chunks are segments of data that represent discrete parts of a larger document or dataset. In Pinecone, each chunk is assigned a unique identifier (ID) to facilitate easy referencing. This structure allows for better organization and retrieval of information, especially in cases where documents contain multiple sections or paragraphs.
Example of Chunks in Action
Imagine you have a lengthy document consisting of several paragraphs. Instead of treating the entire document as a single entity, you can separate it into manageable chunks. This approach helps improve search efficiency and relevance by allowing users to retrieve specific information quickly.
Suggested Reads- 7 Chunking Strategies in RAG You Need To Know
Here’s how you can create and upsert chunks into Pinecone:
from pinecone import Pinecone,ServerlessSpec
from sentence_transformers import SentenceTransformer
# Initialize Pinecone
pc=Pinecone(api_key="YOUR_API_KEY", environment="us-west1-gcp")
# Create a namespace for your data
namespace = "Vector databases"
# Load a pre-trained model for generating embeddings
model = SentenceTransformer('all-MiniLM-L6-v2')
# Sample data representing chunks
documents = [
{"id": "Pinecone", "text": "A fully managed vector database that provides fast, scalable, and high-performance similarity search and retrieval for machine learning models."},
{"id": "Weaviate", "text": "An open-source, schema-based vector database optimized for unstructured data, offering semantic search, modularity, and integration with large language models."},
{"id": "Milvus", "text": "A highly scalable, open-source vector database with robust support for high-dimensional data, used for similarity search and recommendations across diverse domains."}
]
# Generate embeddings for each chunk
for doc in documents:
embedding = model.encode(doc["text"]).tolist()
if "vectordb" not in pc.list_indexes().names():
pc.create_index("vectordb", dimension=len(embedding),metric="cosine",
spec=ServerlessSpec(
cloud='aws',
region='us-east-1'
))
# Upsert chunks to Pinecone
for doc in documents:
pc.Index("vectordb").upsert(vectors=[(doc["id"], embedding)],namespace=namespace)
print("Chunks upserted successfully!")
In this example,Each document is represented as a chunk with an ID and text content, which we then upserted into the specified index.
Embeddings
Embeddings are numerical representations of text, allowing you to transform semantic information into a continuous vector space. This transformation enables machines to understand and process text based on its meaning rather than just its syntactic form. In Pinecone, each chunk can be associated with an embedding that captures its semantic context, making it possible to search for related content effectively.
Generating Embeddings
To generate embeddings, you typically use a pre-trained model from libraries such as Sentence Transformers or OpenAI’s embeddings. Here's how to do it:
from sentence_transformers import SentenceTransformer
# Load a pre-trained model for generating embeddings
model = SentenceTransformer('all-MiniLM-L6-v2')
# Generate embeddings for each chunk
for doc in documents:
embedding = model.encode(doc["text"]).tolist() # Convert to list for upsert
pc.Index("VectorDB").upsert(vectors=[(doc["id"], embedding, namespace)])
In this code snippet, we load a pre-trained Sentence Transformer model and generate embeddings for each chunk of text. The embeddings are then upserted into the Pinecone index, allowing for efficient searching based on the meaning of the text.
Index
An index in Pinecone serves as a structured collection that accepts and stores vector embeddings. It acts as a repository for the embeddings, enabling efficient querying and operations. You can think of an index as a specialized database designed to handle high-dimensional vectors.
Querying an Index
Once you have embeddings stored in an index, you can perform queries to find similar vectors. This process allows you to retrieve relevant chunks based on a given query vector. Here’s how to create an index and perform a query:
# Create an index if it doesn't exist
if "vectordb" not in pc.list_indexes().names():
pc.create_index("vectordb", dimension=len(embedding))
# Querying for similar chunks
query_embedding = model.encode("which is the best vector databases").tolist()
results = pc.Index("VectorDB").query(queries=[query_embedding], top_k=3, namespace=namespace)
print("Query results:", results)
In this example, we first check if the index exists and create it if it doesn't. We then generate a query embedding for a test query and perform a search for the top three most similar chunks in the specified namespace. The results provide insights into which chunks are most relevant to the query.
Namespaces
Namespaces in Pinecone act as logical partitions within an index. They allow you to segment your data into distinct subsets, enabling you to manage and query different datasets independently. Each index can support up to 10,000 namespaces, providing significant flexibility for various applications.
Using Namespaces Effectively
Namespaces are particularly useful when you need to perform operations on different subsets of data without interfering with one another. Here’s how to utilize namespaces in your upsert and query operations:
# Upsert with namespaces
pc.Index("vectordb").upsert(vectors=[("Qdrant", embedding, "vector databases")])
# Query from a different namespace
new_results = pc.Index("vectordb").query(queries=[query_embedding], top_k=3, namespace="vector databases")
print("Query results from new namespace:", new_results)
Returns:
Query results from new namespace:{
"matches": [
{
"id": "Pinecone",
"score": 0.85,
},
{
"id": "Weaviate",
"score": 0.78,
},
{
"id": "Milvus",
"score": 0.76,
} ],
"namespace": "vector databases"
}
In this code snippet, we upsert a new chunk into a different namespace called `new_namespace`. We then perform a query to retrieve results specifically from that namespace, demonstrating how namespaces allow for organized data retrieval.
Conclusion
Pinecone's vector database offers robust features for managing and querying high-dimensional data efficiently. By understanding and leveraging the concepts of chunks, embeddings, indexes, and namespaces, you can build powerful applications that require rapid search and retrieval capabilities.
Whether you're developing recommendation systems, search engines, or natural language processing applications, Pinecone provides the tools you need to succeed. Its structured approach to data organization and retrieval allows you to focus on building intelligent systems without getting bogged down in the complexities of data management.
With Pinecone, you can elevate your AI applications to new heights, making data-driven decisions faster and more effectively.
Frequently Asked Questions?
What is the main purpose of Pinecone Vector Database?
Pinecone helps AI systems organize and find information quickly by storing and managing vector embeddings, making it ideal for search and recommendation systems.
How do chunks work in Pinecone?
Chunks are smaller segments of large documents with unique IDs, making it easier to store and retrieve specific pieces of information efficiently.
What's the difference between indexes and namespaces in Pinecone?
Indexes store all your vector embeddings, while namespaces help organize these vectors into separate groups within an index for better data management.