How to Use React Native FS to Access the Filesystem
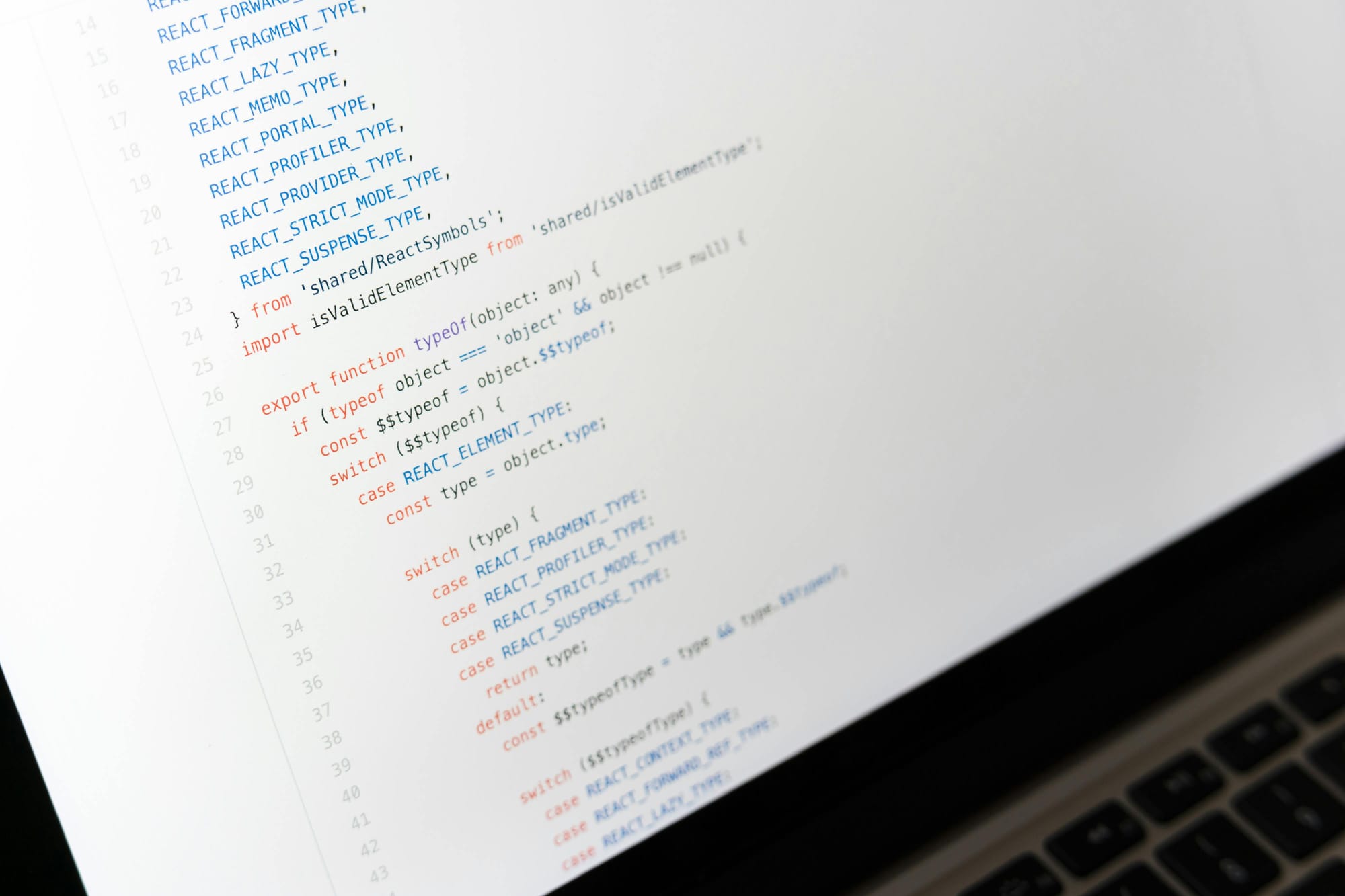
The ability to interact with a device's file system is often crucial. Whether you're building an app that needs to store user data locally, manage downloaded files, or manipulate images, having robust file system access is essential. This is where React Native FS comes into play, providing a powerful solution for developers working with React Native.
React Native FS, often referred to as RNFS, is a comprehensive library that bridges the gap between React Native and the native file systems of iOS and Android. It offers a unified API that allows developers to perform a wide range of file operations, from simple read and write tasks to more complex operations like file downloads, uploads, and directory management.
With React Native FS, you can seamlessly integrate file system capabilities into your app, enhancing its functionality and user experience.
Getting Started with React Native FS
To begin using React Native FS in your project, you first need to install it. You can do this using npm or yarn:
npm install react-native-fs
# or
yarn add react-native-fs
After installation, you'll need to link the library. For React Native versions 0.60 and above, this process is typically automatic. For earlier versions, you might need to run:
react-native link react-native-fs
Now, let's dive into some practical examples of how to use React Native FS.
Reading Files with React Native FS
One of the most common operations is reading files. Here's how you can read the contents of a file:
import RNFS from 'react-native-fs';
const readFile = async () => {
try {
const contents = await RNFS.readFile(RNFS.DocumentDirectoryPath + '/myfile.txt', 'utf8');
console.log(contents);
} catch (error) {
console.error('Error reading file:', error);
}
};
This example demonstrates how to read a text file located in the app's document directory.
Writing Files with React Native FS
Writing files is equally straightforward:
import RNFS from 'react-native-fs';
const writeFile = async () => {
const path = RNFS.DocumentDirectoryPath + '/myfile.txt';
const contents = 'Hello, React Native FS!';
try {
await RNFS.writeFile(path, contents, 'utf8');
console.log('File written successfully!');
} catch (error) {
console.error('Error writing file:', error);
}
};
This code snippet writes a simple string to a file in the document directory.
Downloading Files with React Native FS
React Native FS also provides functionality for downloading files:
import RNFS from 'react-native-fs';
const downloadFile = () => {
const fromUrl = 'https://example.com/myfile.pdf';
const toFile = `${RNFS.DocumentDirectoryPath}/myfile.pdf`;
const options = {
fromUrl: fromUrl,
toFile: toFile,
begin: (res) => {
console.log('Download started');
},
progress: (res) => {
let progressPercent = (res.bytesWritten / res.contentLength) * 100;
console.log(`Downloaded ${progressPercent.toFixed(2)}%`);
},
};
RNFS.downloadFile(options).promise
.then((res) => {
console.log('File downloaded successfully');
})
.catch((error) => {
console.error('Error downloading file:', error);
});
};
This example shows how to download a file, complete with progress tracking.
Managing Directories with React Native FS
React Native FS also allows you to manage directories:
import RNFS from 'react-native-fs';
const createDirectory = async () => {
const path = RNFS.DocumentDirectoryPath + '/MyNewFolder';
try {
await RNFS.mkdir(path);
console.log('Directory created successfully');
} catch (error) {
console.error('Error creating directory:', error);
}
};
This code creates a new directory in the app's document directory.
Checking File Existence with React Native FS
Before performing operations on files, it's often useful to check if they exist:
import RNFS from 'react-native-fs';
const checkFileExists = async () => {
const path = RNFS.DocumentDirectoryPath + '/myfile.txt';
try {
const exists = await RNFS.exists(path);
console.log(`File exists: ${exists}`);
} catch (error) {
console.error('Error checking file existence:', error);
}
};
This function checks whether a specific file exists in the document directory.
Best Practices when Using React Native FS
1. Error Handling: Always wrap your file operations in try-catch blocks to handle potential errors gracefully.
2. Permissions: Be aware of the permissions required for file operations, especially on Android. You may need to request additional permissions for certain operations.
3. Performance: For large files or frequent operations, consider using streams or chunked operations to avoid memory issues.
4. Security: Be cautious about where you read from and write to. Stick to app-specific directories when possible.
5. Cleanup: Remember to clean up temporary files and manage your app's storage usage responsibly.
Conclusion
React Native FS is a powerful tool that opens up a world of possibilities for file system interactions in your React Native apps. From basic read and write operations to more complex file management tasks, it provides a robust and flexible API for all your file system needs.
If you're looking to take your React Native app to the next level with advanced file system capabilities, consider reaching out to F22 Labs. Our team can help you implement efficient file management solutions, optimize your app's performance, and create a seamless user experience. Hire React Native Developers from F22 Labs and let us help you unlock the full potential of your mobile application with our deep expertise in React Native and file system management.
FAQs
Can I use fs in React Native?
No, you can't use Node.js 'fs' module directly in React Native. Instead, use react-native-fs, which provides similar functionality tailored for mobile environments.
What is the encoding of React-Native-fs?
React-Native-fs supports various encodings, with 'utf8' being the default for text files. It also supports 'ascii', 'base64', and others depending on the operation.
Does fs require npm?
Yes, react-native-fs needs to be installed via npm or yarn. It's not part of the core React Native package and requires separate installation and linking.