How to Implement Drag-and-Drop in Flutter?
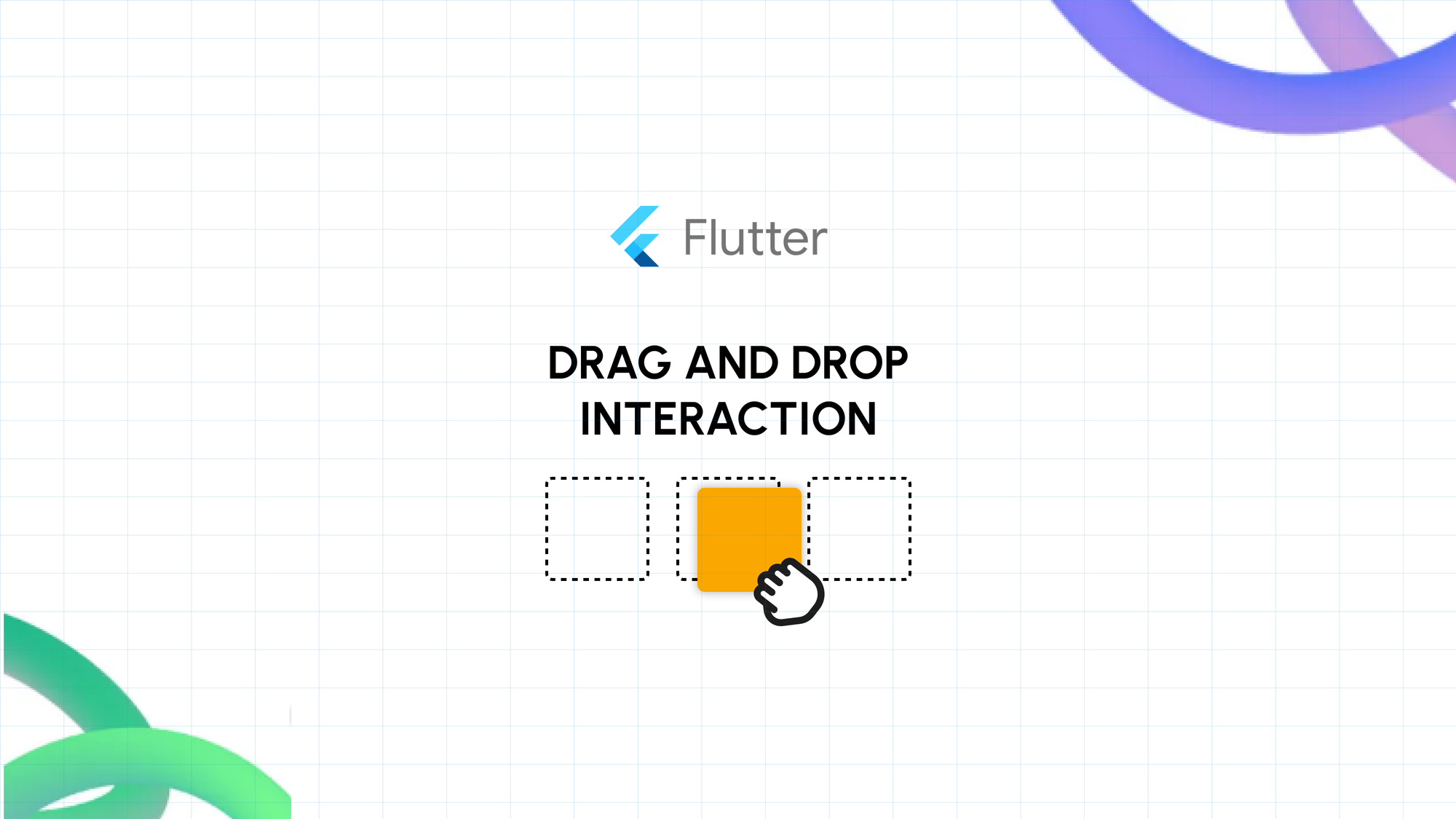
Have you ever marvelled at how smoothly you can rearrange apps on your smartphone's home screen? That's the magic of drag-and-drop at work. As a Flutter developer, you have the power to bring this same intuitive interaction to your apps.
Let's dive into the world of Flutter drag and drop and discover how you can create interfaces that users will love to touch, drag, and interact with.
Why Drag-and-Drop Matters in Modern Apps
Picture this: you're scrolling through a to-do list app, and instead of tapping multiple buttons to change a task's priority, you simply drag it to a new position. Satisfying, right? That's the beauty of drag-and-drop functionality – it makes your app feel alive and responsive.
According to a recent Statista survey, Flutter has taken the mobile development world by storm, with 46% of developers worldwide choosing it as their go-to framework in 2023. With such a growing community, it's no wonder that features like Flutter drag and drop are becoming increasingly important.
Understanding Flutter Drag and Drop
To harness the power of Flutter drag and drop, it's essential to grasp its core components:
- Flutter Draggable: This widget transforms its child into a draggable element, allowing for seamless movement across the screen.
- DragTarget: Serving as a receptacle, this widget is designed to accept and interact with draggable objects.
- LongPressDraggable: A variation of the Draggable widget, activated through a sustained touch, offering an alternative interaction method.
These building blocks form the foundation for creating smooth and intuitive drag-and-drop experiences in Flutter applications.
Implementing Basic Drag and Drop in Flutter
Let's dive into a straightforward implementation of Flutter drag and drop:
import 'package:flutter/material.dart';
class DragDropExample extends StatefulWidget {
@override
_DragDropExampleState createState() => _DragDropExampleState();
}
class _DragDropExampleState extends State<DragDropExample> {
Color caughtColor = Colors.grey;
@override
Widget build(BuildContext context) {
return Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Draggable<Color>(
data: Colors.blue,
child: Container(
width: 100,
height: 100,
color: Colors.blue,
child: Center(child: Text('Draggable')),
),
feedback: Container(
width: 100,
height: 100,
color: Colors.blue.withOpacity(0.5),
child: Center(child: Text('Dragging')),
),
childWhenDragging: Container(
width: 100,
height: 100,
color: Colors.grey,
),
),
DragTarget<Color>(
onAccept: (color) {
setState(() {
caughtColor = color!;
});
},
builder: (context, _, __) {
return Container(
width: 100,
height: 100,
color: caughtColor,
child: Center(child: Text('DragTarget')),
);
},
),
],
);
}
}
This example showcases a simple yet effective implementation of a Flutter Draggable widget that can be dragged onto a DragTarget. Upon successful drop, the target's color transforms, providing visual feedback to the user.
Advanced Drag and Drop Techniques
Creating a Flutter Drag and Drop UI Builder
For more sophisticated applications, developing a Flutter drag and drop UI builder can be a game-changer. This approach is particularly valuable for creating customizable interfaces or workflow editors. Here's a foundational structure for a drag UI builder:
class DragDropUIBuilder extends StatefulWidget {
@override
_DragDropUIBuilderState createState() => _DragDropUIBuilderState();
}
class _DragDropUIBuilderState extends State<DragDropUIBuilder> {
List<Widget> widgets = [];
@override
Widget build(BuildContext context) {
return Column(
children: [
// Palette of draggable widgets
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
DraggableWidget(widget: Text('Text')),
DraggableWidget(widget: Icon(Icons.star)),
DraggableWidget(widget: Container(width: 50, height: 50, color: Colors.blue)),
],
),
// Drop zone
DragTarget<Widget>(
onAccept: (widget) {
setState(() {
widgets.add(widget);
});
},
builder: (context, _, __) {
return Container(
width: 300,
height: 400,
color: Colors.grey[200],
child: Column(children: widgets),
);
},
),
],
);
}
}
class DraggableWidget extends StatelessWidget {
final Widget widget;
DraggableWidget({required this.widget});
@override
Widget build(BuildContext context) {
return Draggable<Widget>(
data: widget,
child: widget,
feedback: widget,
childWhenDragging: Opacity(opacity: 0.5, child: widget),
);
}
}
This Flutter drag and drop UI builder empowers users to craft custom interfaces by dragging widgets from a predefined palette onto a flexible drop zone.
Suggested Reads- Why Do React Native & Flutter Outperform Kotlin & Swift?
Implementing Reorderable Lists
A common application of drag-and-drop functionality is the reordering of list items. Flutter's built-in ReorderableListView widget streamlines this process. Here's an illustrative example:
class ReorderableListExample extends StatefulWidget {
@override
_ReorderableListExampleState createState() => _ReorderableListExampleState();
}
class _ReorderableListExampleState extends State<ReorderableListExample> {
final List<String> _items = List<String>.generate(20, (i) => "Item ${i + 1}");
@override
Widget build(BuildContext context) {
return ReorderableListView(
children: _items.map((item) => ListTile(
key: Key(item),
title: Text(item),
)).toList(),
onReorder: (oldIndex, newIndex) {
setState(() {
if (newIndex > oldIndex) newIndex--;
final String item = _items.removeAt(oldIndex);
_items.insert(newIndex, item);
});
},
);
}
}
This code snippet demonstrates the creation of a dynamic list where users can effortlessly drag and drop the items below into the correct sequence from left to right.
Best Practices for Flutter Drag and Drop
To optimize your Flutter drag and drop implementations, consider these best practices:
- Visual Feedback: Leverage the feedback property of Draggable widgets to provide clear visual cues during drag operations.
- Edge Case Handling: Anticipate and gracefully manage scenarios where users attempt to drag items to invalid locations.
- Performance Optimization: For extensive lists or complex UIs, employ ListView.builder or GridView.builder to ensure smooth performance.
- Accessibility: Design your drag-and-drop interfaces with inclusivity in mind, ensuring they're usable by individuals relying on assistive technologies.
Use Cases for Drag and Drop in Flutter
Drag-and-drop functionality can revolutionize user experience across various app categories:
- Task Management: Enable intuitive task prioritization by allowing users to move items between status columns.
- Photo Organization: Empower users to curate their digital memories by rearranging photos or creating custom albums.
- E-commerce: Streamline the shopping experience with draggable product cards that can be added to a virtual cart.
- Educational Tools: Create engaging learning experiences with interactive quizzes where answers are dragged to their correct positions.
- Music Curation: Allow music enthusiasts to craft the perfect playlist by dragging and reordering tracks.
By integrating Flutter drag and drop in these scenarios, you can craft more intuitive and engaging user interfaces that delight your audience.
Conclusion
Mastering drag-and-drop functionality in Flutter opens up a world of possibilities for creating highly interactive and user-centric applications. From simple list reordering to complex UI builders, Flutter drag and drop capabilities provide developers with the tools to craft truly engaging user experiences.
As you continue to explore and implement drag-and-drop features in your Flutter projects, remember to prioritize user experience, performance optimization, and accessibility. With practice and innovation, you'll be able to create intuitive interfaces that not only meet but exceed user expectations.
Can we do drag and drop in Flutter?
Absolutely! Flutter provides built-in support for drag-and-drop functionality through a suite of widgets including Draggable, DragTarget, and LongPressDraggable. These powerful tools enable developers to implement a wide array of drag-and-drop interactions in their Flutter applications.
How do you implement the drag and drop feature in Flutter?
Implementing drag and drop in Flutter involves three key steps:
- Utilize the Draggable widget to designate items as draggable.
- Create a DragTarget widget to serve as a receptor for dropped items.
- Implement the onAccept callback of the DragTarget to define actions triggered when an item is successfully dropped.
How to drag and drop an image in Flutter?
To enable drag and drop for images in Flutter:
- Encapsulate your Image widget within a Draggable widget.
- Configure both the child and feedback properties of the Draggable to display the Image widget.
- Implement a DragTarget capable of accepting and processing the image data upon drop.
Need a Helping Hand?
At F22 Labs, we're passionate about creating Flutter apps that users love to interact with. Our team of skilled Flutter developers can help you implement advanced drag-and-drop functionality and other interactive features that will set your app apart. Let's work together to bring your app ideas to life – reach out to us today!