How To Implement Dark Mode in Your Flutter App
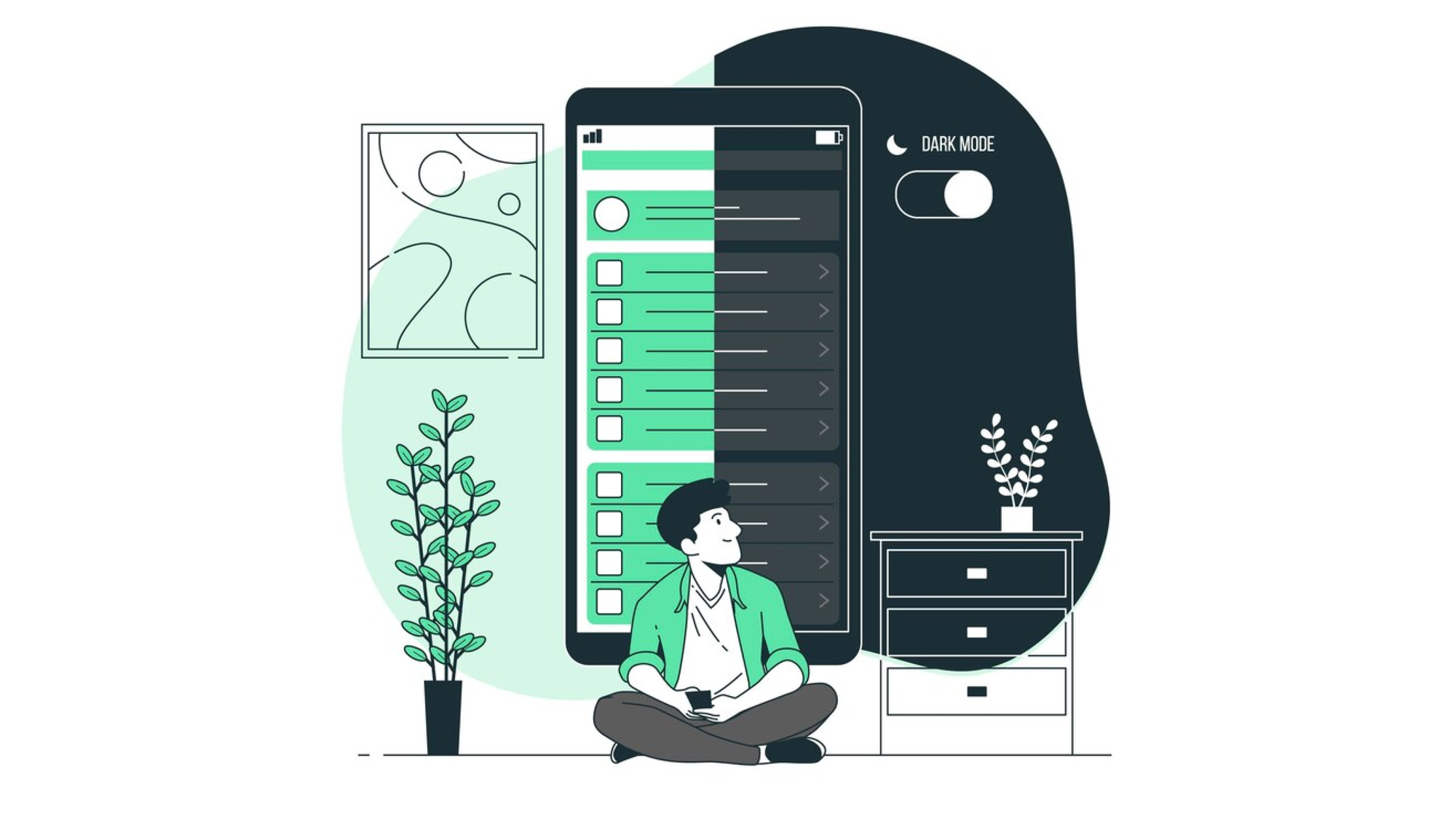
In today's app-driven world, dark mode has become a must-have feature. A 2021 Android Authority survey found that an overwhelming 81.9% of users prefer dark mode in their apps. This trend highlights the need for Flutter developers to incorporate this feature to enhance user satisfaction and stay competitive.
Understanding Theming in Flutter
Flutter theming is the foundation for implementing dark mode. It provides a systematic way to manage your app's visual elements, ensuring consistency and flexibility across your entire application.
The Importance of Flutter Theme
A well-crafted Flutter theme offers multiple benefits:
- Visual cohesion across your app
- Simple customization for branding purposes
- Centralized styling for easier maintenance
- Support for features like dark mode
Implementing Dark Mode in Your Flutter App
Let's break down the process of adding dark mode to your Flutter app.
Step 1: Define Your Flutter Themes
Start by creating distinct themes for light and dark modes in your main.dart file:
import 'package:flutter/material.dart';
final lightTheme = ThemeData(
brightness: Brightness.light,
primaryColor: Colors.blue,
// More light theme properties here
);
final darkTheme = ThemeData(
brightness: Brightness.dark,
primaryColor: Colors.indigo,
// More dark theme properties here
);
Step 2: Set Up Theme Switching
Implement a mechanism to toggle between themes using ChangeNotifier:
import 'package:flutter/material.dart';
class ThemeProvider extends ChangeNotifier {
ThemeMode _themeMode = ThemeMode.light;
ThemeMode get themeMode => _themeMode;
void toggleTheme() {
_themeMode = _themeMode == ThemeMode.light ? ThemeMode.dark : ThemeMode.light;
notifyListeners();
}
}
Step 3: Implement Theme Switching in Your App
Integrate the theme provider into your app structure:
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
void main() {
runApp(
ChangeNotifierProvider(
create: (_) => ThemeProvider(),
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Consumer<ThemeProvider>(
builder: (context, themeProvider, child) {
return MaterialApp(
title: 'Flutter Dark Mode Example',
theme: lightTheme,
darkTheme: darkTheme,
themeMode: themeProvider.themeMode,
home: HomePage(),
);
},
);
}
}
Step 4: Create a Dark Mode Toggle
Add a user-friendly switch to toggle between themes:
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
final themeProvider = Provider.of<ThemeProvider>(context);
return Scaffold(
appBar: AppBar(title: Text('Flutter Dark Mode')),
body: Center(
child: Switch(
value: themeProvider.themeMode == ThemeMode.dark,
onChanged: (_) {
themeProvider.toggleTheme();
},
),
),
);
}
}
This setup allows users to easily switch between dark and light mode in Flutter.
Best Practices for Flutter Dark Theme Implementation
When crafting your Flutter dark theme, keep these guidelines in mind:
- Maintain sufficient color contrast
- Ensure visual consistency across themes
- Respect system-wide theme preferences
- Optimize performance during theme switches
Flutter Dark Theme Colors
Choosing the right colors for your dark theme is crucial:
- Use darker versions of your primary colors
- Avoid pure black for backgrounds
- Ensure text remains easily readable
Look to popular apps like Amazon's Android app dark mode or Procore's dark mode for inspiration.
Detecting System Theme
Enhance user experience by automatically applying the system's theme preference:
import 'package:flutter/material.dart';
class ThemeProvider extends ChangeNotifier {
ThemeMode _themeMode = ThemeMode.system;
ThemeMode get themeMode => _themeMode;
void setThemeMode(ThemeMode mode) {
_themeMode = mode;
notifyListeners();
}
}
This approach allows your app to seamlessly adapt to the user's system-wide theme settings.
Advanced Flutter Dark Mode Techniques
For more complex apps, consider these advanced strategies:
Custom Flutter Dark Theme Colors
Create a custom color palette for more precise control:
import 'package:flutter/material.dart';
class AppColors {
static const Color primaryLight = Colors.blue;
static const Color primaryDark = Colors.indigo;
static const Color backgroundLight = Colors.white;
static const Color backgroundDark = Color(0xFF121212);
// Additional custom colors here
}
Dark Mode Flutter Package
Explore packages like adaptive_theme for additional dark mode functionality in your Flutter app.
Persist Theme Preference
Remember user theme preferences across app sessions:
import 'package:shared_preferences/shared_preferences.dart';
class ThemePreferences {
static const THEME_KEY = "theme_key";
setTheme(bool value) async {
SharedPreferences prefs = await SharedPreferences.getInstance();
prefs.setBool(THEME_KEY, value);
}
getTheme() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
return prefs.getBool(THEME_KEY) ?? false;
}
}
This feature allows users to maintain their preferred theme setting.
Testing Your Flutter Dark Theme
Ensure your dark mode implementation works flawlessly by:
- Testing on various devices and screen sizes
- Verifying compatibility across different OS versions
- Conducting accessibility checks in both themes
- Monitoring performance during theme transitions
Conclusion
Implementing dark mode in your Flutter app is a powerful way to enhance user experience and stay current with design trends. By following these steps and best practices, you can create a seamless and visually appealing dark mode experience.
Remember, Flutter theming extends beyond dark mode, allowing you to create consistent, branded experiences that adapt to various user preferences and system settings.
As you continue developing, explore advanced theming techniques and stay updated on the latest Flutter themes and design trends.
Need expert help?
At F22 Labs, our team of experienced Flutter Developers can assist you in implementing dark mode and other advanced features in your Flutter app. With our expertise in Flutter theming and app development, we can help you create a polished, user-friendly application that stands out in the competitive mobile app market. Reach out to us today to turn your app idea into reality with Flutter.
Frequently Asked Questions?
How do I add dark mode to my Flutter app?
Implement dark mode by defining light and dark ThemeData, using a ThemeProvider with ChangeNotifier, and adding a toggle switch. The system can also automatically detect user preferences.
Can Flutter automatically detect system theme preferences?
Yes, Flutter can detect system theme preferences using ThemeMode.system. This allows your app to automatically switch between light and dark themes based on device settings.
How do I save user theme preferences in Flutter?
Use SharedPreferences to persist theme choices. Store the user's theme selection locally, then retrieve and apply it when the app launches to maintain consistency across sessions.