Create, Export, and Import Components in React Native (vs JAVA)

What is a Component?
The Components are the building blocks in React Native which is similar to the container where all UI elements do accumulate and help to render details in the foreground as a native UI on either Android or iOS devices.
How to create a Component?
I assume that React Native is installed on your machine, If not no worries, Please refer my previous blog post on Environment setup.
import React from 'react';
import { Text, AppRegistry } from 'react-native';
const App = () => (
<Text>Hello Component!</Text>
);
AppRegistry.registerComponent('ComponentDemo', () => App);
Copy-paste the above code snippets into index.js. You’re right to go. Yet confused? Chill! Let’s break the code into pieces in a better way.
React Native JAVA
import React from 'react'; import java.util.*;
import { Text, AppRegistry }
from 'react-native'; import java.text.*;
const App = () => ( public class App {
); }
AppRegistry.registerComponent package com.componentdemo
('ComponentDemo', () => App);
const — This is similar to a class in JAVA, which lets you declare a component block in React Native.
AppRegistry — is the kick starter helps to build React Native apps where all parent or root component should be registered using ‘registerComponent’ function.
Text — This is similar to TextView in Android and Label in iOS.
How to export a Component ?
It’s simple. Create ‘src/components/YOUR_FILE_NAME.js’ inside your project root folder.
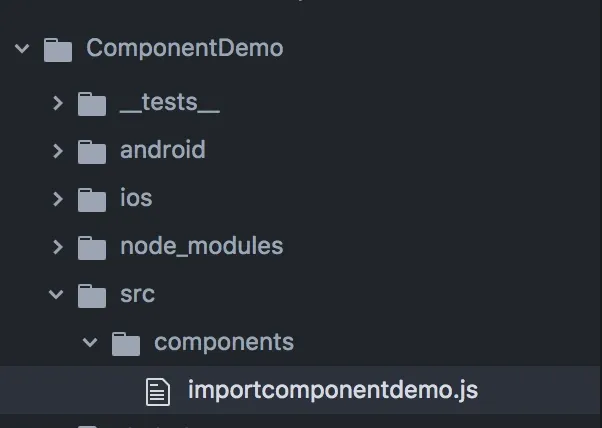
Create a component ‘Title’ and export it as a reusable component.
For Example:
export default Title; //Provides export access for the component
Code Implementation:
import React from 'react';
import { Text } from 'react-native';
const Title = () => (
<Text>Hello Title</Text>
);
export default Title;
How to import a Component from different .js file?
Add the following import statement in the destination Component .js file.
import Title from './src/components/importcomponentdemo';
Complete code:
import React from 'react';
import { AppRegistry } from 'react-native';
import App from './src/components/importcomponentdemo';
const App = () => (
<Title />
);
AppRegistry.registerComponent('ComponentDemo', () => App);
Output:
“Hello Title”
Yes! You’re done. Now it’s possible to access <Title> property from the base file.